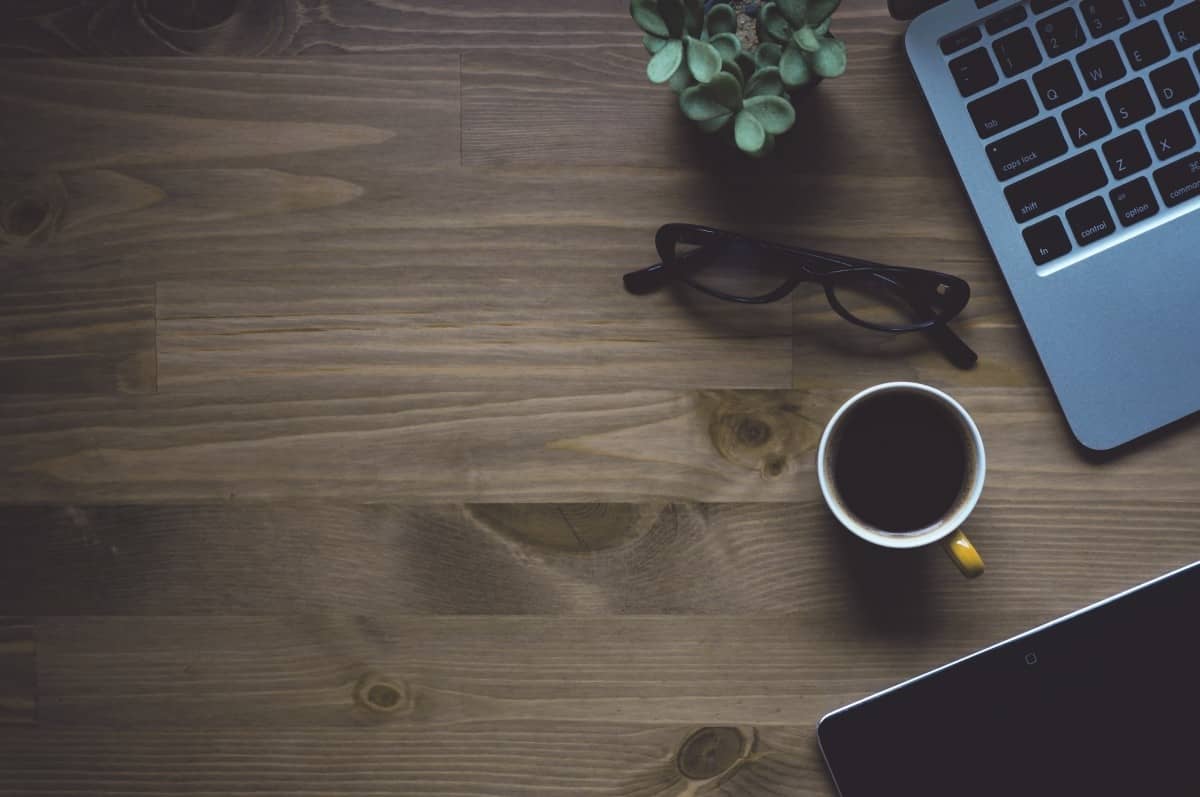
Hey, I'm
Geni Jaho
class ThreadsController extends Controller
{
public function __construct()
{
$this->middleware('auth')->except('index', 'show');
}
/**
* Display a listing of the resource.
*
* @param Channel $channel
* @param ThreadFilters $filters
* @param Trending $trending
* @return Application|Factory|View|Response
*/
public function index(Channel $channel, ThreadFilters $filters, Trending $trending)
{
$threads = $this->getThreads($channel, $filters);
if (request()->wantsJson()) {
return $threads;
}
return view('threads.index', [
'threads' => $threads,
'trending' => $trending->get()
]);
}
/**
* Show the form for creating a new resource.
*
* @return Application|Factory|View
*/
public function create()
{
$channels = Cache::rememberForever('channels', function () {
return Channel::all();
});
return view('threads.create', compact('channels'));
}
/**
* Store a newly created resource in storage.
*
* @param Request $request
* @param SpamFree $spamFree
* @return Application|Redirector|RedirectResponse
*/
public function store(Request $request, SpamFree $spamFree)
{
$request->validate([
'title' => ['required', $spamFree],
'body' => ['required', $spamFree],
'channel_id' => 'required|exists:channels,id'
]);
$thread = Thread::create([
'user_id' => auth()->id(),
'channel_id' => $request->channel_id,
'title' => $request->title,
'body' => $request->body
]);
if ($request->wantsJson()) {
return $thread;
}
return redirect($thread->path())
->with('flash', 'Your thread has been published!');
}
/**
* Display the specified resource.
*
* @param Channel $channel
* @param Thread $thread
* @return Application|Factory|View
* @throws Exception
*/
public function show(Channel $channel, Thread $thread, Trending $trending)
{
if (auth()->check()) {
auth()->user()->read($thread);
}
$trending->push($thread);
return view('threads.show', [
'thread' => $thread,
]);
}
}